Machine Learning for Beginners: Your AI Adventure Begins!
Forget sci-fi fantasies; Machine Learning (ML) is here, and it’s changing the world, right now! Think personalized recommendations, self-driving cars, and even your social media feeds – all powered by clever algorithms. Ready to join the revolution? This beginner’s guide is your passport to understanding and, eventually, doing Machine Learning. Buckle up!
Why Machine Learning? The Big Picture
ML isn’t just a buzzword; it’s a fundamental shift in how we approach problem-solving. Instead of explicitly programming computers with rules, we teach them to learn from data. This ability to adapt and improve over time is what makes it so powerful.
Here’s a quick snapshot of ML’s impact:
Area | Before ML | After ML (Example) |
---|---|---|
Healthcare | Manual diagnosis, drug discovery | AI-powered disease detection, personalized treatments |
Finance | Fraud detection, risk assessment | Automated fraud detection, credit scoring |
Marketing | Generic ads, mass campaigns | Targeted ads, hyper-personalized customer experiences |
Transportation | Human-driven vehicles | Self-driving cars, optimized traffic flow |
The Building Blocks: Essential Concepts
Before you start coding, you need to understand the core ideas. Think of it like learning the rules of the game before playing.
- Data: The fuel of ML! It’s the information we feed into the algorithms. This can be numbers, text, images, anything!
- Algorithms: The recipe. These are the mathematical formulas that allow computers to learn from data and make predictions or decisions.
- Model: The outcome. The result of the algorithm learning from the data. This can then be used to make predictions on new data.
- Training: The learning process. We feed data to the algorithm and adjust it to reduce errors and improve accuracy.
- Prediction: The magic! Using the trained model to make inferences about new, unseen data.
The Machine Learning Family: Meet the Key Players
Just like different branches in a family tree, different types of ML address different problems. Understanding the types will guide your learning path.
-
Supervised Learning: The teacher’s in the room! This is where you provide labeled data (e.g., images labeled “cat” or “dog”). The algorithm learns to map inputs to outputs.
- Use Cases: Spam filtering, image classification, predicting house prices.
-
Unsupervised Learning: No labels needed! The algorithm explores the data to find patterns, clusters, and relationships.
- Use Cases: Customer segmentation, anomaly detection, recommendation systems.
-
Reinforcement Learning: The trial-and-error approach. An agent learns by interacting with an environment and receiving rewards for good actions and penalties for bad ones.
- Use Cases: Game playing (e.g., AlphaGo), robotics, optimizing resource allocation.
Your ML Toolkit: Getting Started
Ready to get your hands dirty? You’ll need a few essential tools.
-
Programming Language: Python reigns supreme! It’s user-friendly, has a massive community, and an enormous library ecosystem for ML.
-
Libraries: These are pre-built sets of code that make your life easier.
- Scikit-learn: A powerhouse for general-purpose ML algorithms.
- TensorFlow / Keras: For deep learning (more on that later!).
- PyTorch: Another excellent deep learning framework.
- Pandas: Data manipulation and analysis.
- NumPy: Numerical computation.
-
Development Environment:
- Jupyter Notebooks: Interactive coding environments, perfect for experimentation and learning.
- Google Colab: A free cloud-based platform with pre-installed ML libraries and GPUs – a HUGE benefit!
- Anaconda: A popular distribution that bundles Python, Jupyter, and many essential libraries.
Your First Machine Learning Project: Hello World!
Let’s get you started with a simple Supervised learning example.
Project: Predicting Iris Flower Species
Goal: Build a model that predicts the species of an iris flower based on its sepal and petal measurements.
Steps:
- Data: We’ll use the classic Iris dataset. You can easily load it using scikit-learn.
- Algorithm: We’ll use a simple algorithm called Logistic Regression.
- Code (Python with scikit-learn):
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn import metrics
# 1. Load the data
iris = load_iris()
X, y = iris.data, iris.target
# 2. Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 3. Create and train the model
model = LogisticRegression(max_iter=200) # Increased max_iter for convergence
model.fit(X_train, y_train)
# 4. Make predictions
y_pred = model.predict(X_test)
# 5. Evaluate the model
print("Accuracy:", metrics.accuracy_score(y_test, y_pred))
Explanation:
- We load the iris dataset.
- We split the data into training and testing sets (essential for evaluating the model’s performance).
- We create a Logistic Regression model and train it using the training data.
- We make predictions on the test data.
- We calculate and print the accuracy of the model.
This is a simplified version, but it demonstrates the basic ML workflow.
Diving Deeper: Where to Go Next
You’ve taken the first steps! Now it’s time to explore and expand your knowledge.
- Online Courses: Platforms like Coursera, edX, Udacity, and Kaggle offer fantastic introductory and advanced ML courses. Look for courses specifically tailored for beginners.
- Books: “Hands-On Machine Learning with Scikit-Learn, Keras & TensorFlow” by Aurélien Géron is an excellent resource.
- Kaggle: A platform for data science competitions. It’s a great place to practice your skills and learn from others.
- Projects: Start small! Work on personal projects using real-world data. This is the best way to solidify your learning.
- Deep Learning: This is a specialized area of ML that uses artificial neural networks with many layers. It’s responsible for some of the most impressive advances in AI, like image recognition and natural language processing. The best way to dive in is by starting with frameworks like TensorFlow or PyTorch.
The Road Ahead: Persistence and Passion
Machine Learning is a challenging but incredibly rewarding field. Be patient, embrace the learning curve, and don’t be afraid to make mistakes. The most successful ML practitioners are those who are curious, persistent, and passionate about solving problems.
So, take the plunge, start exploring, and embark on your own AI adventure! You’ll be amazed at what you can achieve. Good luck, and happy coding!
/images/1.1.419/courses/thumbnails/machine-learning-for-beginners.webp)
Additional Information
Getting Started with Machine Learning for Beginners: A Detailed Guide
This guide provides a comprehensive overview of how to embark on your machine learning journey, breaking down each step into manageable chunks with practical advice and resources.
I. Understanding the Fundamentals:
Before diving into code, it’s crucial to build a solid foundation of fundamental concepts. This will save you time and frustration in the long run.
1. Math Essentials:
- Linear Algebra: This is the bedrock of many machine learning algorithms, especially in areas like dimensionality reduction (e.g., PCA), regression, and neural networks.
- Key Concepts: Vectors, matrices, matrix operations (addition, multiplication, transpose), linear transformations, eigenvalues, eigenvectors.
- Resources:
- Khan Academy’s Linear Algebra Course: https://www.khanacademy.org/math/linear-algebra (Excellent free resource)
- “Linear Algebra Done Right” by Sheldon Axler: A more theoretical but thorough approach.
- MIT OpenCourseWare’s Linear Algebra: https://ocw.mit.edu/courses/mathematics/18-06-linear-algebra-spring-2010/ (Video lectures and notes)
- Calculus: Understanding derivatives and integrals is vital for optimization algorithms (e.g., gradient descent) used to train models.
- Key Concepts: Limits, derivatives, integrals, chain rule, partial derivatives, optimization.
- Resources:
- Khan Academy’s Calculus Course: https://www.khanacademy.org/math/calculus-1 (Again, excellent free resource)
- “Calculus” by James Stewart: A widely-used textbook.
- 3Blue1Brown’s Essence of Calculus series on YouTube: Visual and intuitive explanations.
- Statistics and Probability: Machine learning heavily relies on statistical methods to analyze data, make predictions, and assess model performance.
- Key Concepts: Descriptive statistics (mean, median, standard deviation), probability distributions (normal, binomial, etc.), hypothesis testing, Bayesian inference.
- Resources:
- Khan Academy’s Statistics and Probability Course: https://www.khanacademy.org/math/statistics-probability (Free and excellent for a broad introduction)
- “OpenIntro Statistics” by Diez, Barr, and Çetinkaya-Rundel: Free and comprehensive textbook.
- “Think Stats” by Allen B. Downey (Free online): Focuses on data analysis with Python.
2. Programming Proficiency:
- Python: Python is the dominant language in machine learning due to its rich ecosystem of libraries, ease of use, and readability.
- Key Concepts: Data types (lists, dictionaries, tuples), control flow (if/else, loops), functions, object-oriented programming (classes, objects), working with files.
- Resources:
- Codecademy’s Python course: https://www.codecademy.com/learn/learn-python-3 (Interactive and beginner-friendly)
- Python.org’s official tutorial: https://docs.python.org/3/tutorial/ (Comprehensive and reliable)
- “Automate the Boring Stuff with Python” by Al Sweigart (Free online): Practical guide with real-world examples.
- DataCamp’s Python courses: Focus on data science applications of Python.
- Familiarize yourself with essential Python libraries:
- NumPy: Fundamental package for numerical computing in Python. It provides efficient array operations and mathematical functions.
- Pandas: Powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series, making it easy to clean, explore, and transform data.
- Scikit-learn: The core machine learning library in Python. It provides a wide range of algorithms for classification, regression, clustering, dimensionality reduction, and model selection.
- Matplotlib & Seaborn: Libraries for data visualization. Matplotlib is a general-purpose plotting library, while Seaborn is built on top of Matplotlib and offers higher-level visualizations and statistical plots.
3. Machine Learning Concepts:
- Types of Machine Learning:
- Supervised Learning: The algorithm learns from labeled data (input-output pairs) to make predictions.
- Regression: Predicts a continuous output (e.g., predicting house prices).
- Classification: Predicts a categorical output (e.g., classifying emails as spam or not spam).
- Unsupervised Learning: The algorithm learns from unlabeled data to find patterns, structure, or relationships.
- Clustering: Groups similar data points together (e.g., customer segmentation).
- Dimensionality Reduction: Reduces the number of variables while preserving essential information (e.g., Principal Component Analysis – PCA).
- Reinforcement Learning: An agent learns by interacting with an environment and receiving rewards or penalties. (More advanced; often best approached after mastering supervised and unsupervised learning).
- Supervised Learning: The algorithm learns from labeled data (input-output pairs) to make predictions.
- Key Terminology:
- Features: Input variables used for prediction.
- Labels/Targets: Output variables being predicted.
- Training Data: Data used to train the model.
- Testing Data: Data used to evaluate the model’s performance on unseen data.
- Model: The mathematical representation of the learned patterns.
- Algorithm: The specific procedure used to train the model (e.g., linear regression, decision tree).
- Overfitting: The model learns the training data too well, leading to poor performance on new data.
- Underfitting: The model is too simple to capture the underlying patterns in the data, leading to poor performance.
- Evaluation Metrics: Measures used to assess the model’s performance (e.g., accuracy, precision, recall, F1-score, mean squared error).
- Hyperparameters: Settings that control the model’s learning process (e.g., the learning rate, the number of trees in a random forest).
II. Setting Up Your Environment:
- Choose a Development Environment:
- Local Installation: Install Python and necessary libraries on your computer.
- Anaconda: A popular distribution that includes Python, many essential libraries (NumPy, Pandas, Scikit-learn), and a package manager (conda) for easy installation and management. Highly recommended for beginners.
- Pip: Python’s package installer. Use it to install libraries if not using Anaconda.
pip install numpy pandas scikit-learn matplotlib seaborn
- Cloud-Based Platforms:
- Google Colaboratory (Colab): Free, cloud-based Jupyter Notebook environment with access to GPUs. Excellent for getting started without needing to install anything.
- Kaggle Kernels: Similar to Colab, designed for data science competitions and projects.
- Amazon SageMaker Studio Lab: Free service for machine learning development (requires sign-up).
- Jupyter Notebook/Lab: Web-based interactive environments for creating and running code, visualizing data, and documenting your work. Great for experimentation and exploring data.
- Local Installation: Install Python and necessary libraries on your computer.
- Install Required Libraries: Use
pip
orconda
(if using Anaconda) to install the necessary libraries. For example:# Using conda (Anaconda) conda install numpy pandas scikit-learn matplotlib seaborn # Using pip pip install numpy pandas scikit-learn matplotlib seaborn
III. Learning Through Projects:
Hands-on experience is key. Start with simple projects and gradually increase complexity.
1. Beginner Projects:
- Linear Regression:
- Dataset: Use a simple dataset like the Boston Housing dataset (available in Scikit-learn).
- Goal: Predict house prices based on features like crime rate, number of rooms, and distance to employment centers.
- Steps:
- Load the dataset.
- Explore the data (visualize, understand the features).
- Split the data into training and testing sets.
- Create a linear regression model using
sklearn.linear_model.LinearRegression
. - Train the model on the training data using
model.fit()
. - Make predictions on the testing data using
model.predict()
. - Evaluate the model’s performance using metrics like Mean Squared Error (MSE) or R-squared.
- Classification with Logistic Regression:
- Dataset: The Iris dataset (also in Scikit-learn). This is a classic dataset for classifying different species of iris flowers.
- Goal: Predict the species of an iris based on its sepal and petal measurements.
- Steps: (Similar to linear regression, but use
sklearn.linear_model.LogisticRegression
)- Load the dataset.
- Explore the data.
- Split the data into training and testing sets.
- Create a logistic regression model.
- Train the model.
- Make predictions.
- Evaluate using accuracy, precision, recall, and F1-score.
- K-Nearest Neighbors (KNN) Classification:
- Dataset: Again, use the Iris dataset.
- Goal: Predict the iris species using KNN.
- Steps:
- Load the dataset.
- Explore the data.
- Split the data into training and testing sets.
- Create a KNN model using
sklearn.neighbors.KNeighborsClassifier
. Experiment with different values ofn_neighbors
(the number of neighbors to consider). - Train the model.
- Make predictions.
- Evaluate using accuracy, precision, recall, and F1-score.
2. Intermediate Projects:
- Decision Trees and Random Forests:
- Dataset: Explore datasets from Kaggle or UCI Machine Learning Repository.
- Goal: Build models to predict more complex outcomes, such as customer churn, credit risk, or sentiment analysis.
- Steps:
- Data Cleaning and Preprocessing: Handle missing values, outliers, and categorical features (using techniques like one-hot encoding).
- Feature Engineering: Create new features from existing ones to improve model performance.
- Model Training: Use
sklearn.tree.DecisionTreeClassifier
andsklearn.ensemble.RandomForestClassifier
. Tune hyperparameters (e.g.,max_depth
,n_estimators
) using techniques like cross-validation. - Model Evaluation and Selection: Use more sophisticated evaluation metrics and compare the performance of different models.
- Clustering with K-Means:
- Dataset: Generate synthetic data or find a real-world dataset for customer segmentation or other clustering tasks.
- Goal: Group data points into clusters based on their similarity.
- Steps:
- Load and preprocess data.
- Use
sklearn.cluster.KMeans
to create a K-Means model. - Determine the optimal number of clusters using techniques like the elbow method or silhouette analysis.
- Train the model and visualize the clusters.
- Projects involving time series data:
- Dataset: Consider datasets on stock prices, weather data, sales data, or energy consumption.
- Goals: Perform time-series analysis, forecasting, anomaly detection, and pattern recognition.
- Libraries: You can explore
statsmodels
andprophet
for more advanced time series analysis. - Steps:
- Preprocess the time series data (handling missing values, resampling data to consistent intervals, handling time zones)
- Visualize the time series data.
- Decompose a time series into trend, seasonality, and residual components.
- Model time series data using ARIMA, Exponential Smoothing, or Prophet.
- Evaluate the model’s performance using metrics such as mean absolute error, mean squared error, and root mean squared error.
3. Resources for Projects and Datasets:
- Kaggle: A platform with datasets, code, and competitions. Great for learning from others and competing.
- UCI Machine Learning Repository: A well-known repository of datasets.
- Scikit-learn’s dataset module: Provides access to several built-in datasets for practice.
- DataCamp, Coursera, edX, Udacity: Online learning platforms with structured machine learning courses and projects.
- GitHub: Explore open-source machine learning projects and code. Search for projects related to your interests.
IV. Continuous Learning and Improvement:
Machine learning is a rapidly evolving field. Stay up-to-date by:
- Reading research papers and blogs: Subscribe to newsletters, follow influential researchers on social media, and read articles on Towards Data Science, Analytics Vidhya, and other platforms.
- Taking online courses and attending webinars: Platforms like Coursera, edX, Udacity, and DataCamp offer courses on various machine learning topics.
- Participating in competitions: Kaggle is a great platform to test your skills and learn from other data scientists.
- Building a portfolio: Create a GitHub repository to showcase your projects and code.
- Networking: Connect with other data scientists and machine learning enthusiasts through online communities, meetups, and conferences.
V. Key Considerations and Tips:
- Start small and build incrementally: Don’t try to learn everything at once. Focus on mastering the fundamentals and gradually expanding your knowledge.
- Focus on understanding the concepts: Don’t just memorize code. Understand the underlying principles and why different algorithms work.
- Practice regularly: Consistent practice is essential for solidifying your understanding.
- Don’t be afraid to experiment and make mistakes: Machine learning is an iterative process. You’ll learn a lot from trial and error.
- Ask for help: Don’t hesitate to seek help from online communities (Stack Overflow, Reddit), forums, or mentors.
- Be patient: Learning machine learning takes time and effort. Don’t get discouraged if you don’t understand everything immediately. Keep learning and practicing, and you will improve.
- Choose a path that aligns with your interests: Machine learning has numerous applications. Find a field or problem area that you’re genuinely curious about, and you’ll be more motivated to learn.
- Consider the ethical implications: Machine learning models can have unintended consequences. Be aware of the potential biases in data and the ethical considerations of using machine learning in different applications.
- Don’t be afraid to explore different areas within ML: You don’t need to specialize immediately. Experimenting with different types of models and projects will help you discover your interests and strengths.
- Document your work: Keep a detailed record of your projects, code, and experiments. This will help you learn, track your progress, and showcase your skills.
This detailed guide provides a solid foundation for beginners to navigate the exciting world of machine learning. Remember to stay curious, persistent, and enjoy the journey! Good luck!
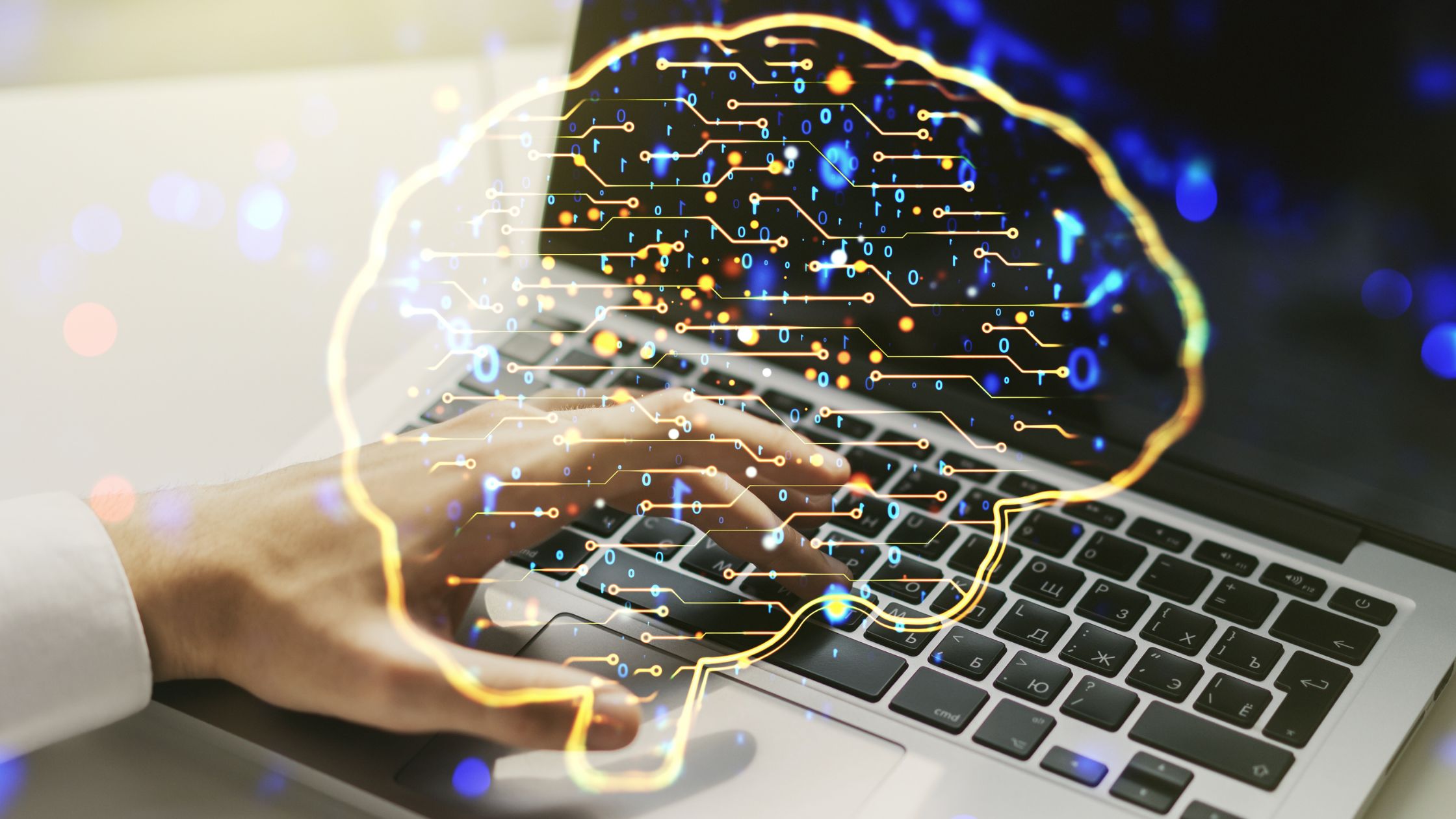